In Java, both the Hashtable and HashMap are data structures based on hashing and implementation of the Map interface the primary distinction is that HashMap is not thread-safe, whereas Hashtable is. As a result, HashMap cannot be used in a multi-threaded Java application without external synchronization. Another distinction is that HashMap supports one null key and null values, whereas Hashtable does not. Furthermore, the hash table achieves thread safety by internal synchronization, which makes it slower than HashMap.
By the way, the distinction between HashMap and Hashtable in Java is frequently addressed in core Java interviews to determine whether the candidate understands the correct usage of collection classes and is aware of alternate alternatives.
This is one of the oldest questions from the Java Collection framework, along with How HashMap Internally Works and ArrayList vs Vector. Hashtable is a legacy Collection class that has been in the Java API for a long time. Still, in Java 4 it was refactored to implement the Map interface, and Hashtable became part of the Java Collection framework.
The subject of Hashtable vs HashMap in Java is so common that it can be found at the top of any list of Java Collection Interview Questions. Before attending any Java programming interview, you cannot afford to study HashMap versus Hashtable.
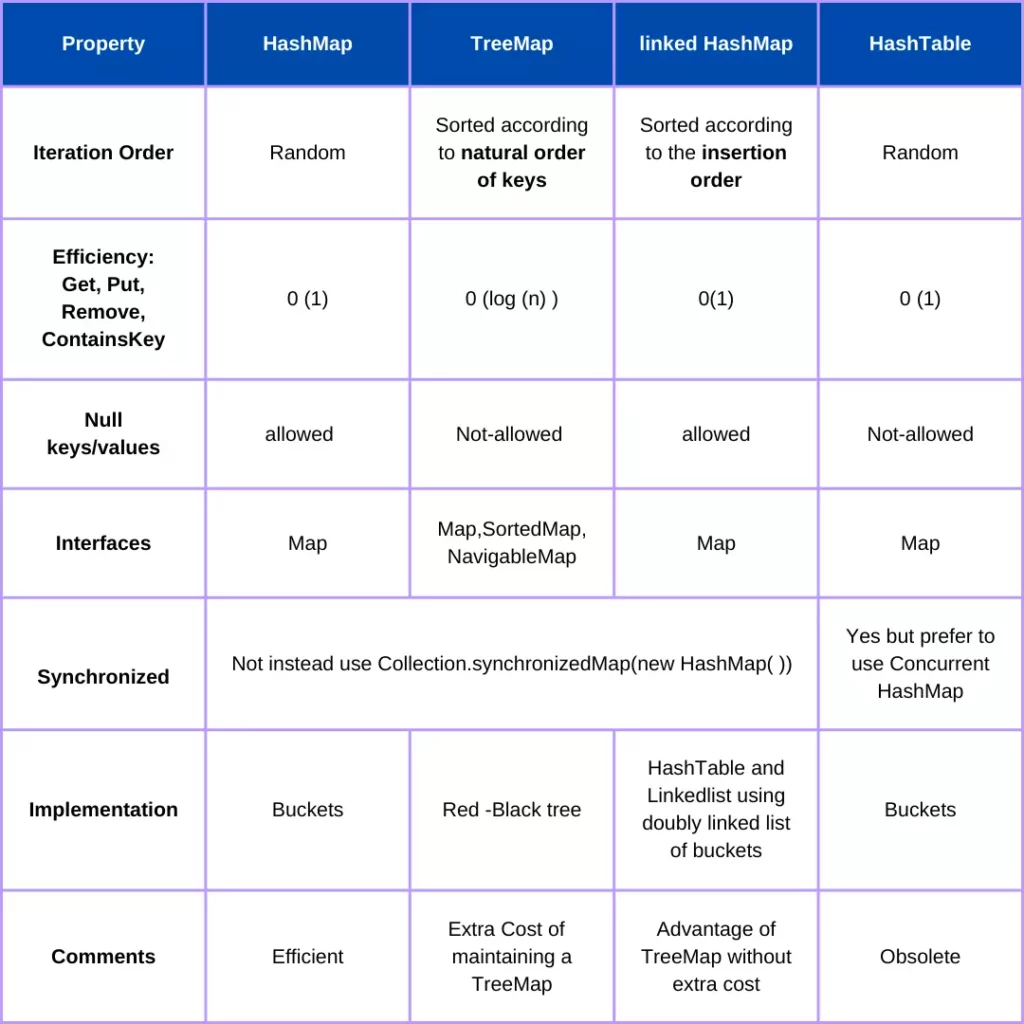
Difference between HashMap and Hashtable in Java
In Java, both HashMap
and Hashtable
are used to store and retrieve key-value pairs. However, there are several differences between them:
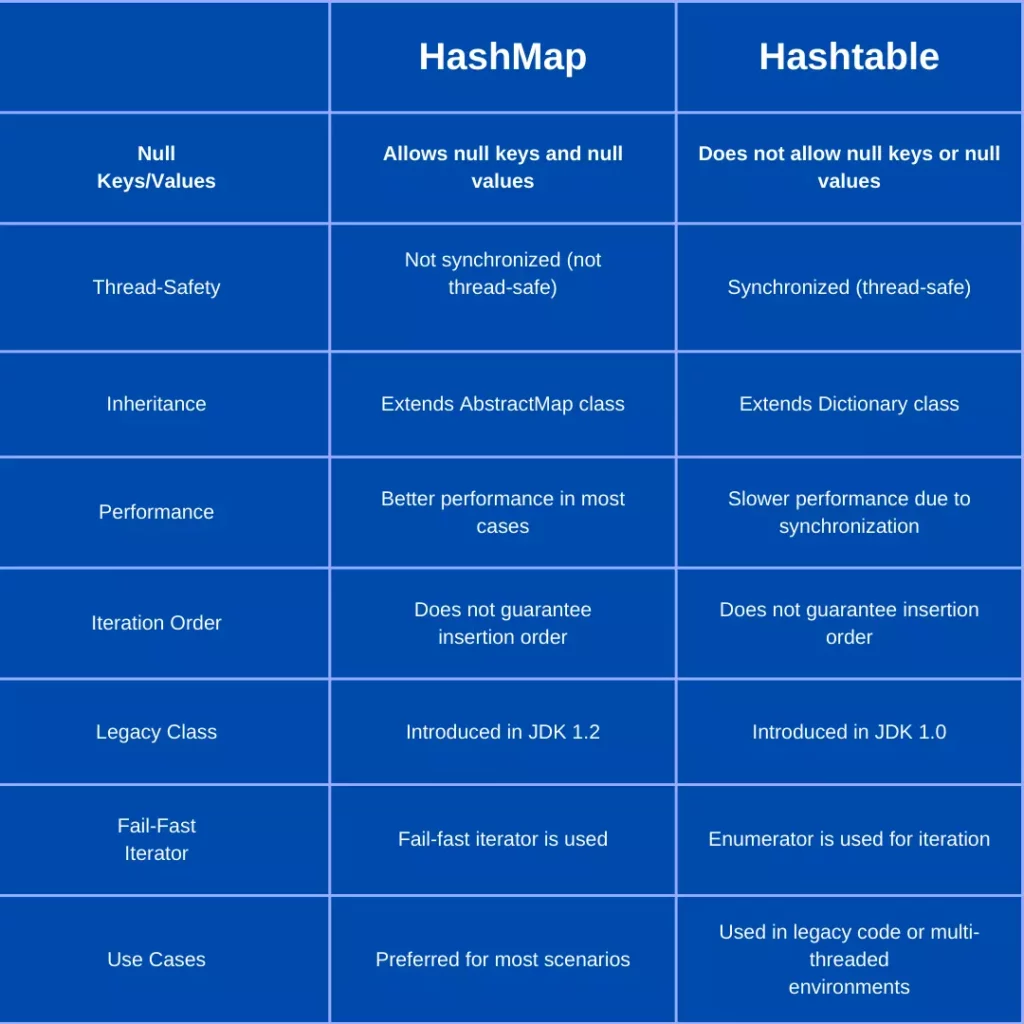
- Synchronization: The most significant difference is that
Hashtable
is synchronized, which means it is thread-safe and can be accessed by multiple threads concurrently without causing data corruption. In contrast,HashMap
is not synchronized and is not thread-safe by default. If you need thread safety withHashMap
, you can use theCollections.synchronizedMap()
method to create a synchronized version. - Null values and keys:
Hashtable
does not allow null keys or values. If you try to store a null key or value, it will throw aNullPointerException
. On the other hand,HashMap
allows a single null key and multiple null values. - Performance:
HashMap
is generally faster thanHashtable
because it does not have the overhead of synchronization. If you do not require thread safety,HashMap
is usually the preferred choice for better performance. - Iterating over elements:
Hashtable
‘s enumerator returns the elements in random order whileHashMap
providing no guarantee regarding the order of elements. If you need a specific iteration order, you can useLinkedHashMap
, which maintains the insertion order. - Inheritance:
Hashtable
is a legacy class and part of the original Java Collections Framework. It extends the obsoleteDictionary
class. On the other hand,HashMap
is a newer class introduced in Java 1.2 as part of the Collections Framework and implements theMap
interface.
Some important terms related to HashMap and Hashtable
Certainly! Here are some important terms related to HashMap
and Hashtable
in Java:
- Key-Value Pair: Both
HashMap
andHashtable
store data in the form of key-value pairs. The key is used to retrieve the corresponding value. - Hashing: Hashing is the process of converting an object into an integer value (hash code) using a hash function.
HashMap
andHashtable
use hashing internally to determine the index or bucket where the key-value pairs are stored. - Hash Code: A hash code is an integer value generated by the hash function for a given object. It is used to calculate the index of the bucket where the key-value pair will be stored in the underlying data structure.
- Bucket: A bucket is a container within the underlying data structure (such as an array or linked list) where the key-value pairs are stored. The index of the bucket is determined by the hash code of the key.
- Collision: Collision occurs when two different keys produce the same hash code, resulting in them being assigned to the same bucket.
HashMap
andHashtable
handle collisions differently. - Chaining: Chaining is a collision resolution technique where each bucket contains a linked list of key-value pairs. If a collision occurs, the new key-value pair is added to the linked list of the corresponding bucket.
- Load Factor: The load factor is a measure of how full the underlying data structure is allowed to get before its capacity is automatically increased. Both
HashMap
andHashtable
have a default load factor of 0.75. - Capacity: The capacity of a hash-based collection is the number of buckets it can hold. It is automatically increased as needed when the load factor is reached. The initial capacity can be specified during the creation of
HashMap
orHashtable
. - Iterator: Both
HashMap
andHashtable
provide an iterator that allows you to iterate over the key-value pairs stored in the collection. The iterator provides methods likenext()
,hasNext()
, andremove()
. - Concurrent Modification Exception: If you modify the underlying collection (e.g., add or remove elements) while iterating over it using an iterator, a
ConcurrentModificationException
may be thrown.
Understanding these terms will help you work effectively with HashMap
and Hashtable
in Java.
Conclusion
In summary, if you need thread safety, require strict non-null values and keys, or are working with legacy code, Hashtable
can be used. However, if you don’t need thread safety, want better performance, and prefer more flexibility, HashMap
is the recommended choice.
- Mog Coin Skyrockets 70% – Discover Why Its Price Could Soar Even Higher!
- How to add live scoreboard in wordpress website
- 36 दिन बीते..कब धरती पर आएंगे दोनों एस्टोनॉट्स? सुनीता विलियम्स-विलमोर ने अंतरिक्ष से बताई स्टारलाइनर की दिक्कतें
- Donald Trump assassination attempt: Barack Obama, Bill Ackman and more extend support, ‘No place for political violence’
- AT&T 2022 security breach hits nearly all cellular customers and landline accounts with contacts